Using Feature Detection, Conditionals, and Groups with Selectors
CSS is designed in a way that allows for relatively seamless addition of new features. Since the dawn of the language, specifications have required browsers to gracefully ignore any properties, values, selectors, or at-rules they do not support. Consequently, in most cases, it is possible to successfully use a newer technology without causing any issues in older browsers.
Consider the relatively new caret-color
property (it changes the color of the cursor in inputs). Its support is still low but that does not mean that we should not use it today.
.myInput {
color: blue;
caret-color: red;
}
Notice how we put it right next to color
, a property with practically universal browser support; one that will be applied everywhere. In this case, we have not explicitly discriminated between modern and older browsers. Instead, we just rely on the older ones ignoring features they do not support.
It turns out that this pattern is powerful enough in the vast majority of situations.
When feature detection is necessary
In some cases, however, we would really like to use a modern property or property value whose use differs significantly from its fallback. In those cases, @supports
comes to the rescue.
@supports
is a special at-rule that allows us to conditionally apply any styles in browsers that support a particular property and its value.
@supports (display: grid) {
/* Styles for browsers that support grid layout... */
}
It works analogously to @media
queries, which also only apply styles conditionally when a certain predicate is met.
To illustrate the use of @supports
, consider the following example: we would like to display a user-uploaded avatar in a nice circle but we cannot guarantee that the actual file will be of square dimensions. For that, the object-fit
property would be immensely helpful; however, it is not supported by Internet Explorer (IE). What do we do then?
Let us start with markup:
<div class="avatar">
<img class="avatar-image" src="..." alt="..." />
</div>
As a not-so-pretty fallback, we will squeeze the image width within the avatar at the cost that wider files will not completely cover the avatar area. Instead, our single-color background will appear underneath.
.avatar {
position: relative;
width: 5em;
height: 5em;
border-radius: 50%;
overflow: hidden;
background: #cccccc; /* Fallback color */
}
.avatar-image {
position: absolute;
top: 50%;
right: 0;
bottom: 0;
left: 50%;
transform: translate(-50%, -50%);
max-width: 100%;
}
You can see this behavior in action here:
See the Pen Demo fallback for object-fit by Jirka Vebr (@JirkaVebr) on CodePen.
Notice there is one square image, a wide one, and a tall one.
Now, if we use object-fit
, we can let the browser decide the best way to position the image, namely whether to stretch the width, height, or neither.
@supports (object-fit: cover) {
.avatar-image {
/* We no longer need absolute positioning or any transforms */
position: static;
transform: none;
object-fit: cover;
width: 100%;
height: 100%;
}
}
The result, for the same set of image dimensions, works nicely in modern browsers:
See the Pen @supports object-fit demo by Jirka Vebr (@JirkaVebr) on CodePen.
Conditional selector support
Even though the Selectors Level 4 specification is still a Working Draft, some of the selectors it defines — such as :placeholder-shown
— are already supported by many browsers. Should this trend continue (and should the draft retain most of its current proposals), this level of the specification will introduce more new selectors than any of its predecessors. In the meantime, and also while IE is still alive, CSS developers will have to target a yet more diverse and volatile spectrum of browsers with nascent support for these selectors.
It will be very useful to perform feature detection on selectors. Unfortunately, @supports
is only designed for testing support of properties and their values, and even the newest draft of its specification does not appear to change that. Ever since its inception, it has, however, defined a special production rule in its grammar whose sole purpose is to provide room for potential backwards-compatible extensions, and thus it is perfectly feasible for a future version to add the ability to condition on support for particular selectors. Nevertheless, that eventuality remains entirely hypothetical.
Selector counterpart to @supports
First of all, it is important to emphasize that, analogous to the aforementioned caret-color
example where @supports
is probably not necessary, many selectors do not need to be explicitly tested for either. For instance, we might simply try to match ::selection
and not worry about browsers that do not support it since it will not be the end of the world if the selection appearance remains the browser default.
Nevertheless, there are cases where explicit feature detection for selectors would be highly desirable. In the rest of this article, we will introduce a pattern for addressing such needs and subsequently use it with :placeholder-shown
to build a CSS-only alternative to the Material Design text field with a floating label.
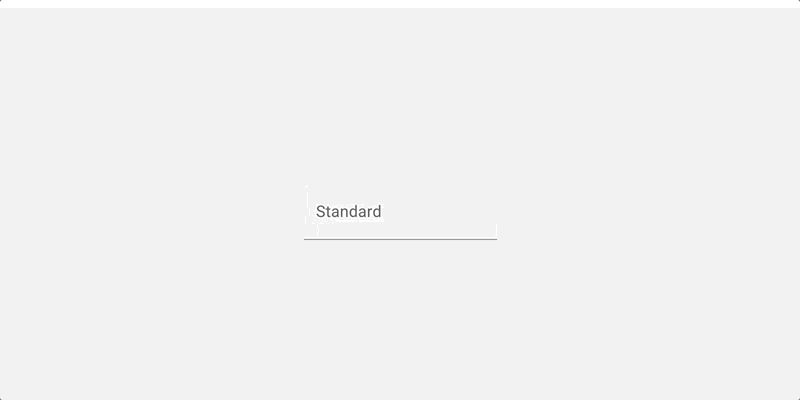
Fundamental property groups of selectors
In order to avoid duplication, it is possible to condense several identical declarations into one comma-separated list of selectors, which is referred to as group of selectors.
Thus we can turn:
.foo { color: red }
.bar { color: red }
…into:
.foo, .bar { color: red }
However, as the Selectors Level 3 specification warns, these are only equivalent because all of the selectors involved are valid. As per the specification, if any of the selectors in the group is invalid, the entire group is ignored. Consequently, the selectors:
..foo { color: red } /* Note the extra dot */
.bar { color: red }
…could not be safely grouped, as the former selector is invalid. If we grouped them, we would cause the browser to ignore the declaration for the latter as well.
It is worth pointing out that, as far as a browser is concerned, there is no difference between an invalid selector and a selector that is only valid as per a newer version of the specification, or one that the browser does not know. To the browser, both are simply invalid.
We can take advantage of this property to test for support of a particular selector. All we need is a selector that we can guarantee matches nothing. In our examples, we will use :not(*)
.
.foo { color: red }
:not(*):placeholder-shown,
.foo {
color: green
}
Let us break down what is happening here. An older browser will successfully apply the first rule, but when processing the the rest, it will find the first selector in the group invalid since it does not know :placeholder-shown
, and thus it will ignore the entire selector group. Consequently, all elements matching .foo
will remain red
. In contrast, while a newer browser will likely roll its robot eyes upon encountering :not(*)
(which never matches anything) it will not discard the entire selector group. Instead, it will override the previous rule, and thus all elements matching .foo
will be green
.
Notice the similarity to @supports
(or any @media
query, for that matter) in terms of how it is used. We first specify the fallback and then override it for browsers that satisfy a predicate, which in this case is the support for a particular selector — albeit written in a somewhat convoluted fashion.
See the Pen @supports for selectors by Jirka Vebr (@JirkaVebr) on CodePen.
Real-world example
We can use this technique for our input with a floating label to separate browsers that do from those that do not support :placeholder-shown
, a pseudo-class that is absolutely vital to this example. For the sake of relative simplicity, in spite of best UI practices, we will choose our fallback to be only the actual placeholder.
Let us start with markup:
<div class="input">
<input class="input-control" type="email" name="email" placeholder="Email" id="email" required />
<label class="input-label" for="email">Email</label>
</div>
As before, the key is to first add styles for older browsers. We hide the label and set the color of the placeholder.
.input {
height: 3.2em;
position: relative;
display: flex;
align-items: center;
font-size: 1em;
}
.input-control {
flex: 1;
z-index: 2; /* So that it is always "above" the label */
border: none;
padding: 0 0 0 1em;
background: transparent;
position: relative;
}
.input-label {
position: absolute;
top: 50%;
right: 0;
bottom: 0;
left: 1em; /* Align this with the control's padding */
z-index: 1;
display: none; /* Hide this for old browsers */
transform-origin: top left;
text-align: left;
}
For modern browsers, we can effectively disable the placeholder by setting its color
to transparent
. We can also align the input
and the label
relative to one other for when the placeholder is shown. To that end, we can also utilize the sibling selector in order to style the label
with respect to the state of the input
.
.input-control:placeholder-shown::placeholder {
color: transparent;
}
.input-control:placeholder-shown ~ .input-label {
transform: translateY(-50%)
}
.input-control:placeholder-shown {
transform: translateY(0);
}
Finally, the trick! Exactly like above, we override the styles for the label
and the input
for modern browsers and the state where the placeholder is not shown. That involves moving the label
out of the way and shrinking it a little.
:not(*):placeholder-shown,
.input-label {
display: block;
transform: translateY(-70%) scale(.7);
}
:not(*):placeholder-shown,
.input-control {
transform: translateY(35%);
}
With all the pieces together, as well as more styles and configuration options that are orthogonal to this example, you can see the full demo:
See the Pen CSS-only @supports for selectors demo by Jirka Vebr (@JirkaVebr) on CodePen.
Reliability and limitations of this technique
Fundamentally, this technique requires a selector that matches nothing. To that end, we have been using :not(*)
; however, its support is also limited. The universal selector *
is supported even by IE 7, whereas the :not
pseudo-class has only been implemented since IE 9, which is thus the oldest browser in which this approach works. Older browsers would reject our selector groups for the wrong reason — they do not support :not
! Alternatively, we could use a class selector such as .foo
or a type selector such as foo
, thereby supporting even the most ancient browsers. Nevertheless, these make the code less readable as they do not convey that they should never match anything, and thus for most modern sites, :not(*)
seems like the best option.
As for whether the property of groups of selectors that we have been taking advantage of also holds in older browsers, the behavior is illustrated in an example as a part of the CSS 1 section on forward-compatible parsing. Furthermore, the CSS 2.1 specification then explicitly mandates this behavior. To put the age of this specification in perspective, this is the one that introduced :hover
. In short, while this technique has not been extensively tested in the oldest or most obscure browsers, its support should be extremely wide.
Lastly, there is one small caveat for Sass users (Sass, not SCSS): upon encountering the :not(*):placeholder-shown
selector, the compiler gets fooled by the leading colon, attempts to parse it as a property, and when encountering the error, it advises the developer to escape the selector as so: :not(*):placeholder-shown
, which does not look very pleasant. A better workaround is perhaps to replace the backslash with yet another universal selector to obtain *:not(*):placeholder-shown
since, as per the specification, it is implied anyway in this case.
The post Using Feature Detection, Conditionals, and Groups with Selectors appeared first on CSS-Tricks.